Ahsin Irshad
Senior Flutter Developer | Ex. Native Android Developer
Vibration In Flutter. Learn how to implement OnTap Vibration… | Feb, 2024
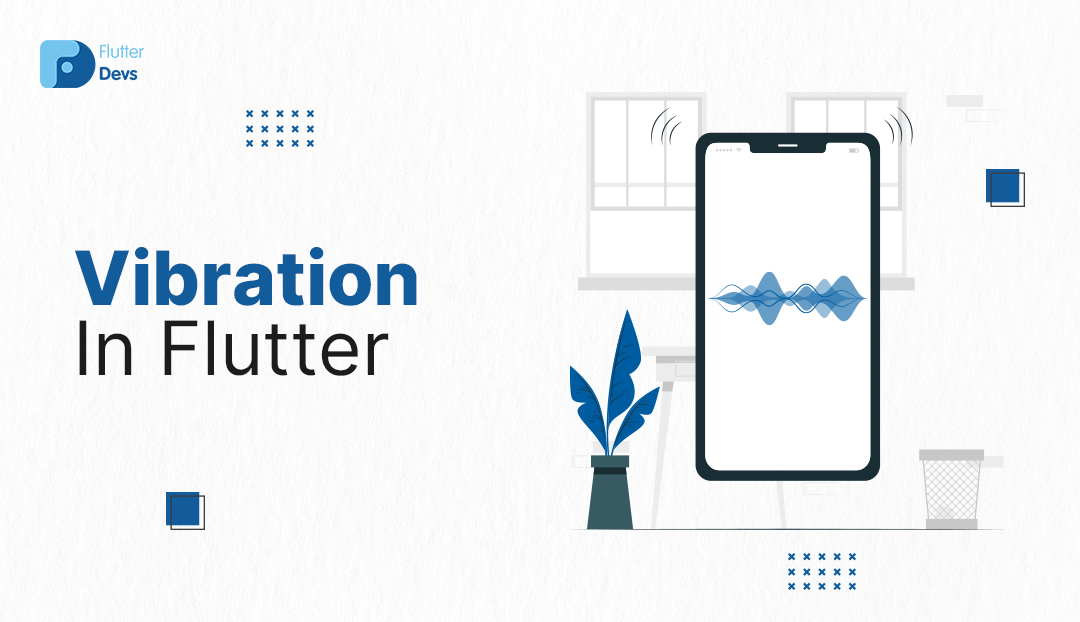
Step 1: Add the dependencies
Add dependencies to pubspec — yaml file.
dependencies:
flutter:
sdk: flutter
vibration: ^1.8.4
Step 2: Import
import 'package:vibration/vibration.dart';
Step 3: Run flutter packages get in the root directory of your app.
Step 4: Add Vibration permission
Add the Vibration permission in
android/app/src/main/AndroidManifest.xml
:
<uses-permission android:name="android.permission.VIBRATE" />
Methods Of Vibration Package:
- > hasVibrator — This method is utilized to check if the vibrator is available on the device.
- > hasAmplitudeControl — This method is utilized to check if the vibrator has amplitude control in your device.
- > hasCustomVibrationsSupport — This method is utilized to check if the device can vibrate with a custom duration, custom pattern, or custom intensities.
In the body, we will add the three ElevatedButtons() wrapped to it container. On the first button, we will add the default vibrate. But the default vibrate was 500ms and I was added 100ms. Also, we will check if the device has a vibration feature. On this second button, we will set the duration of the vibration was 2 sec means 2000ms.
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text("Vibrate Default"),
onPressed: () async {
if (await Vibration.hasVibrator() != null) {
Vibration.vibrate(duration: 100);
}
},
),
),
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text("Vibrate For 2 Sec"),
onPressed: () {
Vibration.vibrate(duration: 2000);
},
),
),
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text("Vibrate With Pattern"),
onPressed: () {
Vibration.vibrate(pattern: [500, 1000, 500, 2000, 500, 3000]);
},
),
)
On the third button, we will vibrate with a pattern that means wait then vibrate again and repeat, etc. 500ms was waited, 1000ms vibrated, 500ms was waited, 2000ms, etc.
When we run the application, we ought to get the screen’s output like the underneath screen capture.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_vibration_demo/splash_screen.dart';
import 'package:vibration/vibration.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const Splash(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
automaticallyImplyLeading: false,
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () {
HapticFeedback.heavyImpact();
},
child: const Text(“Vibrate Using HapticFeedback”)),
const SizedBox(
height: 20,
),
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text(“Vibrate Default”),
onPressed: () async {
if (await Vibration.hasVibrator() != null) {
Vibration.vibrate(duration: 100);
}
},
),
),
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text(“Vibrate For 2 Sec”),
onPressed: () {
Vibration.vibrate(duration: 2000);
},
),
),
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(5),
child: ElevatedButton(
child: const Text(“Vibrate With Pattern”),
onPressed: () {
Vibration.vibrate(pattern: [500, 1000, 500, 2000, 500, 3000]);
},
),
)
],
),
),
);
}
}
In the article, I have explained the Vibration in Flutter; you can modify this code according to your choice. This was a small introduction to the Vibration in Flutter User Interaction from my side, and it’s working using Flutter.
I hope this blog will provide you with sufficient information on Trying Vibration in your Flutter projects. We will show you what the Introduction is. Make a demo program for working on the Vibration using the HapticFeedback class and vibration package in your Flutter applications. So please try it.
❤ ❤ Thanks for reading this article ❤❤
If I need to correct something? Let me know in the comments. I would love to improve.
Clap 👏 If this article helps you.
#Vibration #Flutter #Learn #implement #OnTap #Vibration #Feb