Ahsin Irshad
Senior Flutter Developer | Ex. Native Android Developer
Moving Marker Using Polyline On Google Maps In Flutter | Feb, 2024
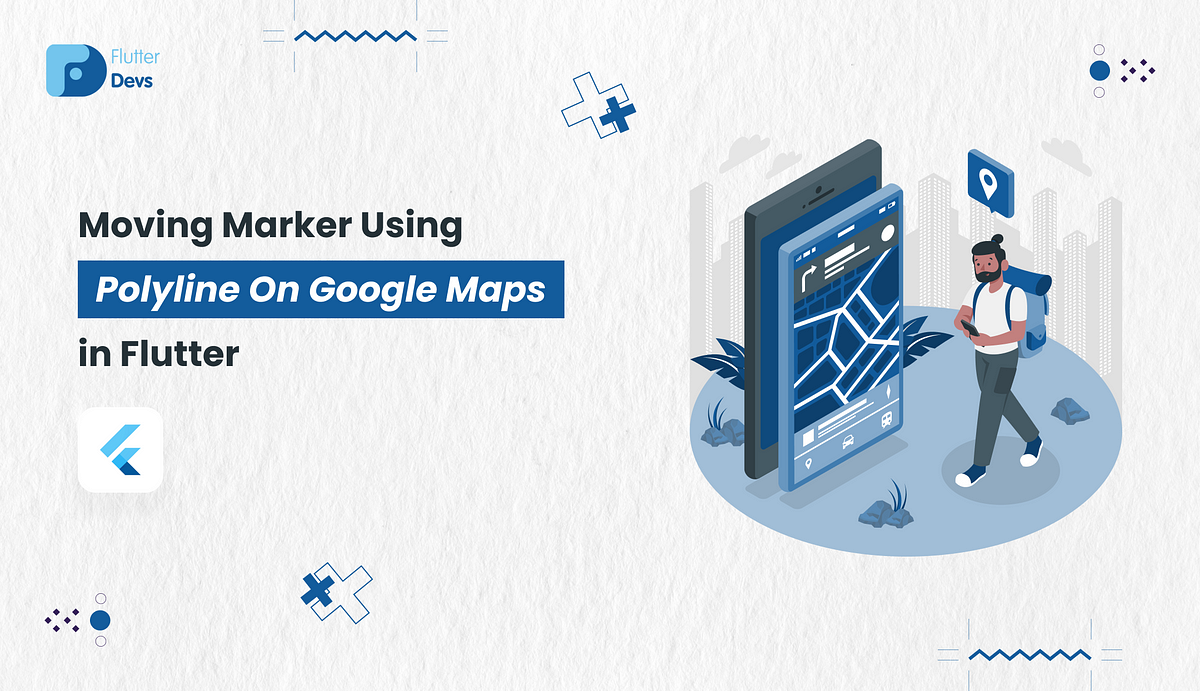
Step 1: Add the dependencies
Add dependencies to pubspec — yaml file.
dependencies:
flutter:
sdk: flutter
google_maps_flutter: ^2.5.2
Step 2: Import
import 'package:google_maps_flutter/google_maps_flutter.dart';
Step 3: Run flutter packages get in the root directory of your app.
Step 4: Update the build.gradle File
Set the
minSdkVersion
inandroid/app/build.gradle
:
android {
defaultConfig {
minSdkVersion 20
}
}
Step 5: Add API Key
For Andriod:
Add the Api key in android/app/src/main/AndroidManifest.xml
:
<manifest ...
<application ...
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_API_KEY_HERE"/>
</application>
</manifest>
For Ios:
To set up, specify your API key in the application delegate ios/Runner/AppDelegate.m
:
import GoogleMaps
...
GMSServices.provideAPIKey("YOUR_API_KEY_HERE")
You need to implement it in your code respectively:
Create a new dart file called
main.dart
inside thelib
folder.
In the main .dart file. We will create a new class MyHomePage(). In this class, we will define a GoogleMapControlle:r as a mapController variable and Set Polylines as a polylines variable ie equal to a curly bracket.
GoogleMapController? mapController;
Set<Polyline> polylines = {};
Now, we will set the marker variable was markers are equal to the Set(). Also, we will add an initial current location was available loc1 is equal to the LatLng().
Set<Marker> markers = Set();
LatLng loc1 = const LatLng(28.612898, 77.365930);
Then, we will add the two position variables pos1 and pos2 of the polylines.
late LatLng pos1;
late LatLng pos2;
Now, we will add the initState() method. In this method, we will add the initial position of the moving marker was the position is equal to loc1.latitude, loc1.longitude in square bracket. Also, the pos1 and pos2 variable is equal to the loc1. in this method, we will add the addMarkers() method. We will define the below code.
@override
void initState() {
position = [
loc1.latitude,
loc1.longitude
]; //initial position of moving marker
pos1 = loc1;
pos2 = loc1;
addMarkers();
super.initState();
}
We will create addMarkers() method. In this method, we will add marderId, position, and icon.
addMarkers() async {
markers.add(Marker(
markerId: MarkerId(loc1.toString()),
position: loc1,
icon: BitmapDescriptor.defaultMarker));
setState(() {
});
}
We will create an animation() method. In this method, start the moving marker. Another, method we will create is movingMarker(). We will dine the below code.
animation(result) {
i = 0;
deltaLat = (result[0] - position[0]) / numDeltas;
deltaLng = (result[1] - position[1]) / numDeltas;
movingMarker();
}
movingMarker() {
position[0] += deltaLat;
position[1] += deltaLng;
var latlng = LatLng(position[0], position[1]);
markers = {
Marker(
markerId: const MarkerId(“moving marker”),
position: latlng,
icon: BitmapDescriptor.defaultMarker,
)
};
pos1 = pos2;
pos2 = LatLng(position[0], position[1]);
polylines.add(Polyline(
polylineId: PolylineId(pos2.toString()),
visible: true,
width: 5,
//width of polyline
points: [
pos1,
pos2,
],
color: Colors.blue, //color of polyline
));
setState(() {
});
if (i != numDeltas) {
i++;
Future.delayed(Duration(milliseconds: delay), () {
movingMarker();
});
}
}
In the body part, we will add the GoogleMap() method. In this method, we will add zoomGesturesEnabled was true, set the initialCameraPosition as zoom is 14, and the target was your loc1 wrap to CameraPosition(), add variable on polylines, map type was normal.
GoogleMap(
zoomGesturesEnabled: true,
initialCameraPosition: CameraPosition(
target: loc1,
zoom: 14.0,
),
markers: markers,
polylines: polylines,
mapType: MapType.normal,
onMapCreated: (controller) {
//method called when map is created
setState(() {
mapController = controller;
});
},
)
onMapCreated was this method called when a map is created. Inside onMapCreated, we will add the setState() function. In this function, we will add mapController is equal to the controller. We will add the variable markers on markers.
Now, we will create a FloatingActionButton(). In this button, we will add backgroundColor was red.shade300, its child adds the text “Start Moving”.
floatingActionButton: SizedBox(
height: MediaQuery.of(context).size.height * 0.3,
width: MediaQuery.of(context).size.width * 0.175,
child: FloatingActionButton(
backgroundColor: Colors.red.shade300,
child: const Center(
child: Text(
"Start\nMoving",
textAlign: TextAlign.center,
)),
onPressed: () {
var result = [28.6279, 77.3749];
//latitude and longitude of new position
animation(result);
//start moving marker
},
),
),
onPressed we will add the latitude and longitude of the new position if was var result is equal to the [28.6279, 77.3749] also add animation(result) for the start moving marker.
When we run the application, we ought to get the screen’s output like the underneath screen capture.
#Moving #Marker #Polyline #Google #Maps #Flutter #Feb