Ahsin Irshad
Senior Flutter Developer | Ex. Native Android Developer
Flutter’s Integration with Native Platforms | Jan, 2025
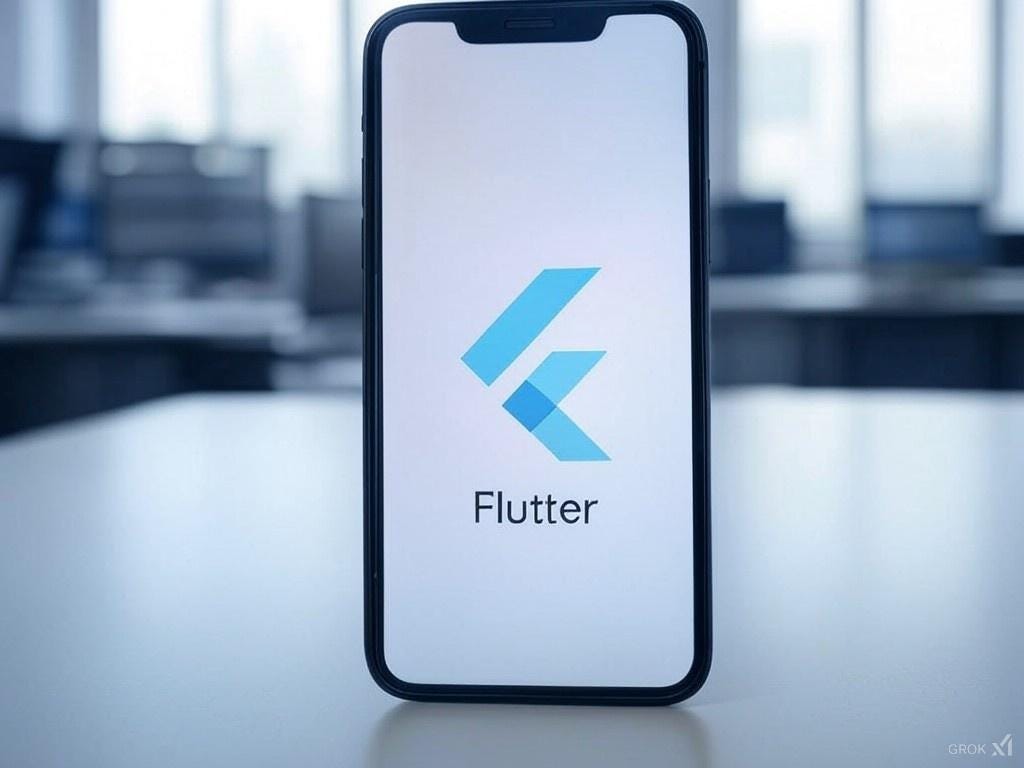
One of Flutter’s biggest strengths is its ability to integrate with native platforms, allowing developers to leverage platform-specific functionalities along- side Flutter’s cross-platform capabilities. This is done using Platform Channels and Dart FFI (Foreign Function Interface). Today, we’ll take a deeper dive into Platform Channels 👇
Platform Channels are a key feature in Flutter that connect Dart code with native platform code. They allow Flutter apps to communicate with the underlying platform — whether it’s Android, iOS, macOS, Linux, or Windows. This communication is essential for accessing native features like cameras, GPS, sensors, and other platform-specific functionalities not directly available through the Flutter framework, including third-party SDKs not built for Flutter.
Platform Channels in Flutter provide three main methods
- Method Channel
Used for asynchronous method calls from Flutter to the native side. The app sends a method call, and the native code executes a specific function, returning the result.
Use Case: Fetching the current battery level or retrieving device information. - Event Channel
Designed for creating a data stream from the native platform to Dart. It’s ideal for continuous or periodic data, such as sensor readings or GPS updates.
Use Case: Subscribing to real-time location changes or sensor data updates. - BasicMessage Channel
Allows simple, asynchronous message exchanges between Dart and platform-specific code. UnlikeMethodChannel
, it’s better suited for sending and receiving raw messages using different data formats, including JSON and binary.
Use Case: Transmitting custom data structures between Flutter and native code.
Let’s walk through a step-by-step example of using Method Channel in Flutter. We’ll create a simple app where the Flutter (Dart) code communicates with the native platform (Android) to fetch the device’s battery level.
Step 1: Define the Method Channel in Flutter (Dart)
In your Flutter app, you need to import package:flutter/services.dart
and define a Method Channel.
// The name must be the same in the native implementation
static const platform = MethodChannel('com.theflutterway.battery/methods');
String _batteryLevel = ‘Unknown battery level.’;
Future _getBatteryLevel() async {
String batteryLevel;
try {
final result = await platform.invokeMethod(‘getBatteryLevel’);
batteryLevel = ‘Battery level at $result % .’;
} on PlatformException catch (e) {
batteryLevel = “Failed to get battery level: ‘${e.message}’.”;
}
setState(() {
_batteryLevel = batteryLevel;
});
}
Just call the method when the button is pressed and display the result in a text widget.
Step 2: Implement the Android-specific code
Open the MainActivity.kt file located in the kotlin
folder. If your project uses Java, open the MainActivity.java file located in the java
folder instead. By default, Flutter uses Kotlin, so we’ll demonstrate with that for now. For Java check the official doc
Inside the configureFlutterEngine()
method, create a MethodChannel and call setMethodCallHandler()
. Be sure to use the same channel name that was used on the Flutter client side.
import androidx.annotation.NonNull
import io.flutter.embedding.android.FlutterActivity
import io.flutter.embedding.engine.FlutterEngine
import io.flutter.plugin.common.MethodChannel
class MainActivity: FlutterActivity() {
private val CHANNEL = “com.theflutterway.battery/methods”
override fun configureFlutterEngine(@NonNull flutterEngine: FlutterEngine) {
super.configureFlutterEngine(flutterEngine)
MethodChannel(flutterEngine.dartExecutor.binaryMessenger, CHANNEL).setMethodCallHandler {
call, result ->
// This method is invoked on the main thread.
// TODO
}
}
}
Then, add the necessary imports at the top of the file:
import android.content.Context
import android.content.ContextWrapper
import android.content.Intent
import android.content.IntentFilter
import android.os.BatteryManager
import android.os.Build.VERSION
import android.os.Build.VERSION_CODES
Now, add the following method in the MainActivity
class, below the configureFlutterEngine()
method:
private fun getBatteryLevel(): Int {
val batteryLevel: Int
if (VERSION.SDK_INT >= VERSION_CODES.LOLLIPOP) {
val batteryManager = getSystemService(Context.BATTERY_SERVICE) as BatteryManager
batteryLevel = batteryManager.getIntProperty(BatteryManager.BATTERY_PROPERTY_CAPACITY)
} else {
val intent = ContextWrapper(applicationContext).registerReceiver(null, IntentFilter(Intent.ACTION_BATTERY_CHANGED))
batteryLevel = intent!!.getIntExtra(BatteryManager.EXTRA_LEVEL, -1) * 100 / intent.getIntExtra(BatteryManager.EXTRA_SCALE, -1)
}
return batteryLevel
}
Finally, let’s complete the setMethodCallHandler()
method that we added earlier. Replace TODO
with the following 👇
MethodChannel(flutterEngine.dartExecutor.binaryMessenger, CHANNEL).setMethodCallHandler {
// This method is invoked on the main thread.
call, result ->
if (call.method == "getBatteryLevel") {
val batteryLevel = getBatteryLevel()
if (batteryLevel != -1) {
result.success(batteryLevel)
} else {
result.error(“UNAVAILABLE”, “Battery level not available.”, null)
}
} else {
result.notImplemented()
}
}
Now rebuild
the App and you are done ✅ 🥳
Thanks for reading, let me know how can make content better.
#Flutters #Integration #Native #Platforms #Flutter #Jan